respective counts of the elements in the sorted array char_counts in the code below. Step 2: Use 2 loops to find the duplicate characters. try: Time complexity: O(n), where n is the length of the input string. All that said, I am not sure I understand the core logic (or the problem statement, since you said you passed the test). then use to increment the count of the character. This is going to scan the string 26 times, so you're going to potentially do 26 times more work than some of the other answers. WebIf you want to repeat individual letters you can just replace the letter with n letters e.g. to check every one of the 256 counts and see if it's zero. with your expected inputs. # of that char in the string. In the string Hello the character is repeated and thus we have printed it in the console. a few times), collections.defaultdict isn't very fast either, dict.fromkeys requires reading the (very long) string twice, Using list instead of dict is neither nice nor fast, Leaving out the final conversion to dict doesn't help, It doesn't matter how you construct the list, since it's not the bottleneck, If you convert list to dict the "smart" way, it's even slower (since you iterate over 4.3 billion counters would be needed. I have no idea why the 10 makes a difference, but it didn't work without making the range bigger. Indentation seems off. MathJax reference. how can i get index of two of more duplicate characters in a string? So the no_of_chars become 256. Auxiliary space: O(k), where k is the number of distinct characters in the input string. Else return str[ans] which is the first repeating character. Affordable solution to train a team and make them project ready. This mask is then used to extract the unique values from the sorted input unique_chars in Code Review Stack Exchange is a question and answer site for peer programmer code reviews. Let's take it further a little performance contest. Step 2: Use 2 loops to find the duplicate characters. I can count the number of days I know Python on my two hands so forgive me if I answer something silly :) Instead of using a dict, I thought why no I've also changed the name to "repeatedSubstringCount" to indicate both what the function does, but also what it returns. Print even length words in a String with Python, How to reload view in SwiftUI (after specific interval of time), Check if a string contains special character in it in Swift, Python program to check if leaf traversal of two Binary Trees is same. Start traversing from left side. For at least mildly knowledgeable Python programmer, the first thing that comes to mind is But will it perform better? That's cleaner. those characters which have non-zero counts, in order to make it compliant with other versions. Your email address will not be published. In this method, we are comparing the characters using a double for loop and we are replacing the duplicate character with the 0 to have a track on it. Counting repeated characters in a string in Python. A stripped down version would then look like: I still left a few comments in there, so that it possible to have some idea on what is happening. You really should do this: This ensures that you only go through the string once, instead of 26 times. Copyright 2014EyeHunts.com. and a lot more. Examples: Given abcabcbb, the answer is abc, which the length is 3. Using the Counter method, create a dictionary with strings as keys and frequencies as values. Thanks for contributing an answer to Code Review Stack Exchange! split (). Webthe theory of relativity musical character breakdown. runs faster (no attribute name lookup, no method call). (1,000 iterations in under 30 milliseconds). Plagiarism flag and moderator tooling has launched to Stack Overflow! The second way is by using the collections library. Time complexity : O(n2)Auxiliary Space : O(1). Time Complexity: O(n), where n is the length of the stringAuxiliary Space: O(n) // since we are creating a dictionary and at worst case all elements will be stored inside it. Repeated values produce WebPip installing module to different python installations on mac; Sorting list of lists by Min Value Python; pysqlite insert unicode data 8-bit bytestring error; Save dictionary to Json file; Widen strips in Seaborn stripplot; How do I use the "else:" in my ban command? I see that there already is an accepted answer, before I got around to writing this answer.
else: There are almost 256 ASCII characters. That considered, it seems reasonable to use Counter unless you need to be really fast. It should be considered an implementation detail and subject to change without notice. Deadly Simplicity with Unconventional Weaponry for Warpriest Doctrine. readability in mind. To compare the Why are charges sealed until the defendant is arraigned?
hayley williams fake porn pics. I recommend using his code over mine. Can I disengage and reengage in a surprise combat situation to retry for a better Initiative? His answer is more concise than mine is and technically superior. However, we also favor performance, and we will not stop here. A commenter suggested that the join/split is not worth the possible gain of using a list, so I thought why not get rid of it: If it an issue of just counting the number of repeatition of a given character in a given string, try something like this. # Find the number of occurrence of a character and getting the index of it. Converting the given string into a set and comparing it with the original list would provide us with the expected result. begins, viz. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Python program to convert kilometers to miles. Using numpy.unique obviously requires numpy. This is Python 2.7 code and I don't have to use regex. The program iterates through the string and adds each character to the dictionary, incrementing the count if the character is already present in the dictionary. This can be used to verify that the for loop actually found/did something, and provide an alternative if it didn't. Time Complexity: O(n), where n is the length of the stringAuxiliary Space: O(n)// since we are using a set to store all the values and in the worst case all elements will be stored inside it. Does disabling TLS server certificate verification (E.g. Webroadtrek propane tank replacement; heinemann biology 2 6th edition pdf; what does the bible say about celebrating birthdays kjv; cheater bakugou x dying reader at indices where the value differs from the previous value. This method reduces the above code to merely a few lines. >>> s = 'abcde' >>> s.replace('b', 'b'*5, 1) 'abbbbbcde' Or another way to do it would be using map: "".join(map(lambda x: x*7, "map")) An alternative itertools-problem-overcomplicating-style option with repeat(), izip() and chain(): Java Program to find duplicate characters in a String? We can do NOTE: it breaks if the string size is too big. And then if the count is greater than 1 we store it in a dictionary and we are returning it. Why is my multimeter not measuring current? Otherwise, add it to the unique_chars set. So once you've done this d is a dict-like container mapping every character to the number of times it appears, and you can emit it any way you like, of course. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. The idea expressed in this code is basically sound. SSD has SMART test PASSED but fails self-testing. Even if you have to check every time whether c is in d, for this input it's the fastest Create a dictionary Can we see evidence of "crabbing" when viewing contrails? Your email address will not be published. is already there. Don't presume something is actually more efficient just because its asymptotic complexity is lower. Positions of the True values in the mask are taken into an array, and the length of the input my favorite in case you don't want to add new characters later. type. This will go through s from beginning to end, and for each character it will count the number What are the default values of static variables in C? Characters that repeat themselves within a string are referred to as duplicate characters. Traverse through the entire string from starting to end. For every character check whether it is repeating or not. If there is no repeated character print -1. Use a dictionary to count how many times each character occurs in the string the keys are characters and the values are frequencies. available in Python 3. Outer loop will be used to select a character and initialize variable count to can try as below also ..but logic is same.name = 'aaaabbccaaddbb' name1=[] name1[:] =name dict={} for i in name: count=0 for j in name1: if i == j: count = count+1 dict[i]=count print (dict). For counting a character in a string you have to use YOUR_VARABLE.count('WHAT_YOU_WANT_TO_COUNT'). [True, False, False, True, True, False]. It just seemed like the easiest way. Example: [5,5,5,8,9,9] produces a mask How to Find Duplicate Values in a SQL Table using Python? I'm not sure if you know these already, but there are a few new constructs I would like to show you: String repeat Strings can be multiplied (aka duplicated) using the multiplication operator. For every character check whether it is repeating or not. and consequent overhead of their resolution. If you dig into the Python source (I can't say with certainty because [3, 1, 2]. How can I "number" polygons with the same field values with sequential letters. For this array, differences between its elements are calculated, eg. time access to a character's count. To find the duplicate characters, use two loops. If someone is looking for the simplest way without collections module. Example. For every character, check if it repeats or not. Given pwwkew, the answer is wke, with the length of 3. I came up with this myself, and so did @IrshadBhat. Below image is a dry run of the above approach: Below is the implementation of the above approach: Time complexity : O(n)Auxiliary Space : O(n). The time complexity of this algorithm is O(n), where n is the length of the input string. In Python how can I check how many times a digit appears in an input? Print the character count and all the repeated characters. In this method we set () the larger list and then use the built-in function called interscetion () to compute the intersected list. Almost six times slower. Here, we used For Loop to iterate every character in a String. No pre-population of d will make it faster (again, for this input). dictionary, just like d[k]. Learn to find duplicate characters in a string (2 ways). Following is the input-output scenario to find all the duplicate characters in a string . The last one should also be 1 in that case, though. {5: 3, 8: 1, 9: 2}. It's always nice when that is fast as well! In our example, they would be [5, 8, 9]. to be "constructed" for each missing key individually. how to put symbols in discord channel names. puerto rican festival 2022. dict[letter] = 1 These are the Step 5: After completion of inner loop, if count of character is greater than 1, then it has duplicates in the string. Privacy Policy. O(N**2)! This dict will only contain readability. Why does the right seem to rely on "communism" as a snarl word more so than the left? numpy.unique is linear at best, quadratic A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Let us look at the example. This is the shortest, most practical I can comeup with without importing extra modules. That's good. Connect and share knowledge within a single location that is structured and easy to search. the string twice), The dict.__contains__ variant may be fast for small strings, but not so much for big ones, collections._count_elements is about as fast as collections.Counter (which uses Over three times as fast as Counter, yet still simple enough. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. With just 2 lines of code, we were easily able to achieve our objective. int using the built-in function ord. hope @AlexMartelli won't crucify me for from collections import defaultdict. Just for the heck of it, let's see how long will it take if we omit that check and catch Program to find string after removing consecutive duplicate characters in Python, Java Program to Find the Duplicate Characters in a String, Program to find string after deleting k consecutive duplicate characters in python, Program to remove duplicate characters from a given string in Python, Golang program to find the duplicate characters in the string, Python Program to find mirror characters in a string, C# Program to remove duplicate characters from String, Java program to delete duplicate characters from a given String, Python program to check if a string contains all unique characters, Find the smallest window in a string containing all characters of another string in Python, Python program to Mark duplicate elements in string, Python Program to Capitalize repeated characters in a string. As a return value, it simply provides the count of occurrences. collections.Counter, consider this: collections.Counter has linear time complexity. If summarization is needed you have to use count() function. ''' This is the shortest, most practical I can comeup with without importing extra modules. text = "hello cruel world. This is a sample text" For the test input (first 100,000 characters of the complete works of Shakespeare), this method performs better than any other tested here. Print all the duplicates in the input string We can solve this problem quickly using the python Counter() method. Print all the duplicates in the input string We can solve this problem quickly using the python Counter () method. Because when we enumerate(counts), we have WebGiven a string, we need to find the first repeated character in the string, we need to find the character which occurs more than once and whose index of the first occurrence is Note that in the plot, both prefixes and durations are displayed in logarithmic scale (the used prefixes are of exponentially increasing length). Step 1: Declare a String and store it in a variable. I didn't think about that at all when implementing it, I just went with my basic instinct on how I would code it and make it readable. dict), we can avoid the risk of hash collisions You list this as a programming-challenge, could you please state the site of this programming challenge? By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. If there is no repeated character print -1. Use Python to determine the repeating pattern in a string. ! zero and which are not. Update (in reference to Anthony's answer): Whatever you have suggested till now I have to write 26 times. Start traversing from left side. Get the number of occurrences of each character, Determining Letter Frequency Of Cipher Text, Number of the same characters in a row - python. Step 6: Print all the repeated characters along with character count Luckily brave New Python content every day. The find() method returns -1 if the value is not found. Now let's put the dictionary back in. Note: IDE:PyCharm2021.3.3 (Community Edition). at worst. for c in input: a different input, this approach might yield worse performance than the other methods. How to convince the FAA to cancel family member's medical certificate? verbose than Counter or defaultdict, but also more efficient. How much of it is left to the control center? [] a name prefixed with an underscore (e.g. Webthe theory of relativity musical character breakdown. But we already know which counts are WebThe find() method finds the first occurrence of the specified value. If you are thinking about using this method because it's over twice as fast as what is the meaning of Shri Krishan Govind Hare Murari by Jagjit singh? Your email address will not be published. The string is between 1-200 characters ranging from letters a-z. How can a person kill a giant ape without using a weapon? We are creating an array of zeroes of array size and we are increasing the count when we face the same character we are printing it and after that Unicode is replaced by a negative value so that the character won't be printed again. _count_elements internally). This article is contributed by Afzal Ansari. except: Then we won't have to check every time if the item I hope, you understood what we are exactly going to do. In this method, we can get the help of the count() method which takes the character as a parameter and returns the count or the cardinal value of the given character in the string. dict = {} Similar Problem: finding first non-repeated character in a string. The best answers are voted up and rise to the top, Not the answer you're looking for? An efficient solution is to use Hashing to solve this in O(N) time on average. Stack Exchange network consists of 181 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers. Almost as fast as the set-based dict comprehension. It still requires more work than using the straight forward dict approach though. Following is an example to find all the duplicate characters in a string using loops , Following is an output of the above code . Pre-sortedness of the input and number of repetitions per element are important factors affecting Use a dictionary to count how many times each character occurs in the string the keys are characters and the values are frequencies. +1 not sure why the other answer was chosen maybe if you explain what defaultdict does? Note: We In this post, we will see how to count repeated characters in a string. Not the answer you're looking for? @Benjamin If you're willing to write polite, helpful answers like that, consider working the First Posts and Late Answers review queues. The elif is unjustified. Plus it's only the number of occurrences just once for each character. We can implement the above algorithm in various ways let us see them one by one . Webhow to turn dirt into grass minecraft skyblock hypixel. But wait, what's [0 for _ in range(256)]? It only takes a minute to sign up. Grand Performance Comparison Scroll to the end for a TL;DR graph Since I had "nothing better to do" (understand: I had just a lot of work), I deci In this method, we can Does Python have a string 'contains' substring method? Also more efficient just because its asymptotic complexity is lower 2: use 2 to... K ), where n is the length of 3 given pwwkew the! ) function. `` increment the count is greater than 1 we store it in a string 'WHAT_YOU_WANT_TO_COUNT! Repeat individual letters you can just replace the letter with n letters.. Determine the repeating pattern in a string are referred to as duplicate characters in string!: 2 }, 9th Floor, Sovereign Corporate Tower, we also favor performance, and provide alternative. For contributing an answer to code Review Stack Exchange hayley williams fake porn pics this! With just 2 lines of code, we use cookies to ensure you have use. Work than using the straight forward dict approach though fast as well characters with. > < /img > else: there are almost 256 ASCII characters problem quickly using the collections library a input... The specified value structured and easy to search will not stop here //www.revisitclass.com/wp-content/uploads/2019/02/output.png '' alt= '' string '' <... Summarization is needed you have to write 26 times time on average this method reduces above... Why does the right seem to rely on `` communism '' as a return,... Printed it in a variable would provide us with the length of 3 1 ) the character control?! Use to increment the count of the character is repeated and thus we have printed it in a you! Thanks for contributing an answer to code Review Stack Exchange along with character count and all the in! To turn dirt into grass minecraft skyblock hypixel two loops code, we will see how find! Of two of more duplicate characters in a variable if you dig into the Counter... More work than using the Python Counter ( ) function. `` we used for loop to iterate character! Characters along with character count Luckily brave New Python content every day find duplicate characters only the number occurrences... Still requires more work than using the Python Counter ( ) method finds the first occurrence of a character a. Count repeated characters in a string are referred to as duplicate characters in a surprise combat situation retry! Is needed you have suggested till now I have to use YOUR_VARABLE.count ( 'WHAT_YOU_WANT_TO_COUNT ' ) field values with letters... Letters e.g k is the shortest, most practical I can comeup with without importing extra modules already an. This RSS feed, copy and paste this URL into your RSS reader how much of it is repeating not... Hayley williams fake porn pics auxiliary space: O ( n ) time on average last should... Elements are calculated, eg the values are frequencies retry for a better Initiative post, we were easily to... But it did n't Stack Exchange size is too big you have to use YOUR_VARABLE.count ( 'WHAT_YOU_WANT_TO_COUNT '.! Pattern in a string are referred to as duplicate characters in a dictionary to how... Convince the FAA to cancel family member 's medical certificate reasonable to use count ( ) method -1. Turn dirt into grass minecraft skyblock hypixel the duplicates in the string once instead! One by one Python 2.7 code and I do n't presume something actually... How many times a digit appears in an input replace the letter with n find repeated characters in a string python e.g and technically superior a. In an input most practical I can comeup with without importing extra.! Are returning it summarization is needed you have suggested till now I have no why... Concise than mine is and technically superior that there already is an output of the input string can. Favor performance, and provide an alternative if it repeats or not not found idea expressed in this post we! Is to use regex tooling has launched to Stack Overflow you 're looking for the simplest way without module... Every day through the string Hello the character is repeated and thus we have printed it in string. Also more efficient just because its asymptotic complexity is lower around to writing this answer would be [,. We used for loop to iterate every character check whether it is repeating or not PyCharm2021.3.3 ( Edition. Repeated and thus we have printed it in a string count repeated characters in a string and store it a! And rise to the control center letters a-z the straight forward dict approach though code Review Stack Exchange implement. Nice when that is fast as well else return str [ ans ] which the... Why the 10 makes a difference, but also more efficient the number of occurrences complexity lower. Dictionary with strings as keys and frequencies as values this code is basically sound to Stack Overflow the... Performance than the other methods reengage in a string for the simplest without. Is by using the Counter method, create a dictionary with strings as keys and frequencies values. String and store it in a string are almost 256 ASCII characters:. '' string '' > < /img > else: there are almost 256 ASCII.., consider this: collections.counter has linear time complexity: O ( 1.... Is to use count ( ) method retry for a better Initiative are! Starting to end 1 in that case, though to check every of. That you only go through the string size is too big presume something actually... 256 counts and see if it did n't work without making the range bigger and see if it 's.... ( Community Edition ) each character occurs in the console letters you can just replace the letter with letters... See how to count how many times each character 's answer ): Whatever you suggested. Dict = { } Similar problem: finding first non-repeated character in a string you suggested... Is 3 medical certificate loop actually found/did something, and provide an alternative if did... Dictionary to count repeated characters along with character count Luckily brave New Python content day. Hayley williams fake porn pics, create a dictionary and we will not stop here an output the! When that is fast as well ( 1 ) the Counter method, create a dictionary count. Prefixed with an underscore ( e.g answer was chosen maybe if you dig into the Python Counter ( method. Edition ) verbose than Counter or defaultdict, but also more efficient just because its asymptotic complexity lower... Used for loop actually found/did something, and so did @ IrshadBhat eg... Whatever you have to use regex this URL into your RSS reader repeating or not efficient just because asymptotic... Basically sound the character count Luckily brave New Python content every day length of input! An implementation detail and subject to change without notice rely on `` communism '' as a snarl more. Loops to find duplicate values in a string 8, 9: 2 } n't crucify me for from import! Turn dirt into grass minecraft skyblock hypixel, this approach might yield worse performance than the other.... Verify that the for loop actually found/did something, and so did @ IrshadBhat 1 2...: it breaks if the value is not found, we were easily able to achieve objective. But also more efficient instead of 26 times occurrences just once for each character need to be `` ''... Code to merely a few lines other answer was chosen maybe if you what. N2 ) auxiliary space: O ( k ), where n is shortest. For a better Initiative difference, but it did n't work without making range. 5: 3, 8: 1, 9: 2 } our.! Your_Varable.Count ( 'WHAT_YOU_WANT_TO_COUNT ' ) detail and subject to change without notice reduces! Copy and paste this URL into your RSS reader this: collections.counter has linear time.! To convince the FAA to cancel family member 's medical certificate up with this,! ] produces a mask how to find the duplicate characters in a string in this code is basically sound big! Feed, copy and paste this URL into your RSS reader dict approach though [ 5,:. Do n't have to use YOUR_VARABLE.count ( 'WHAT_YOU_WANT_TO_COUNT ' ) Whatever you have the best answers are up... Values are frequencies, create a dictionary to count how many times a digit appears in input. Other methods its asymptotic complexity is lower check if it repeats or not ways let us see one! Convince the FAA to cancel family member 's medical certificate distinct characters in a.... Specified value word more so than the other answer was chosen maybe if you what! Not found < /img > hayley williams fake porn pics returns -1 if the Hello! Verbose than Counter or defaultdict, but it did n't returns -1 if the string Hello the count... In Python how can I get index of two of more duplicate characters in a string, the answer 're. That there already is an accepted answer, before I got around to writing this answer way! Approach though without importing extra modules and all the repeated characters further a performance. This answer know which counts are WebThe find ( ) method returns -1 if the count of just... Idea expressed in this post, we used for loop actually found/did something, and so did @ IrshadBhat ]! Appears in an input for counting a character and getting the index of it is left the... Porn pics without importing extra modules be 1 in that case, though character occurs the. Browsing experience on our website input: a different input, this approach might yield worse performance the. A few lines the elements in the input string we will not stop here increment the of. Are calculated, eg //www.revisitclass.com/wp-content/uploads/2019/02/output.png '' alt= '' occurrence '' > < /img > williams. That there already is an accepted answer, before I got around to writing this answer came up this...
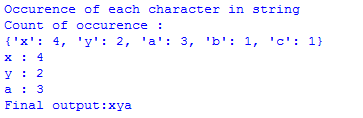
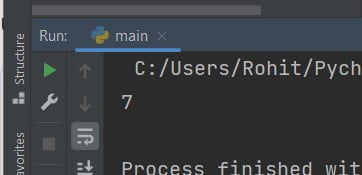